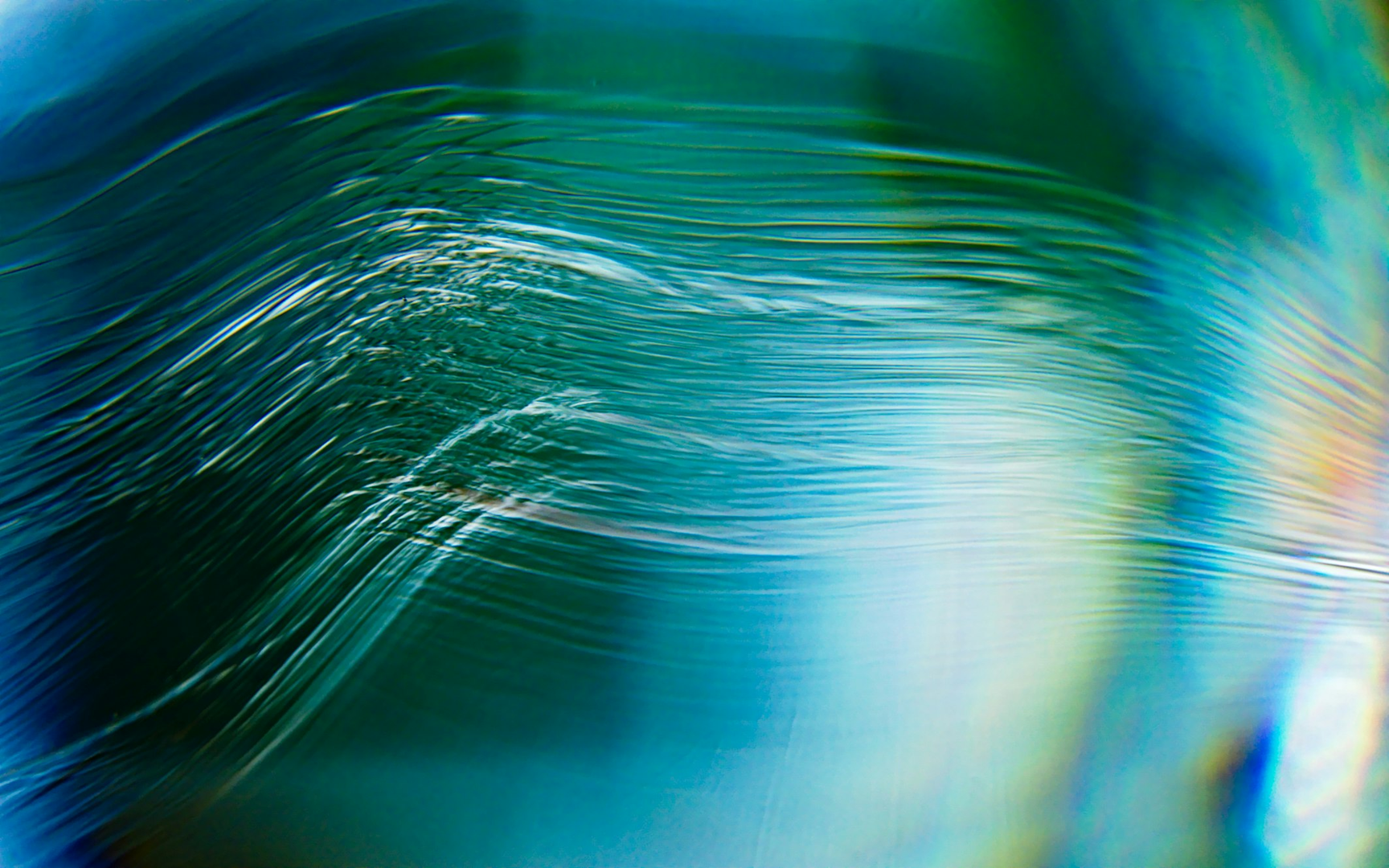
I can still vividly recall the day when I confidently crafted a piece of code utilizing Java Streams, brimming with the belief that I had fully conquered their intricacies. The code appeared pristine, streamlined, and sophisticated — that is, until an unforeseen bug took our production system by storm.
When Code Elegance Became Deceptive
In the community of Java developers, I, like many, cherished the fluency that Streams brought to coding. On one particular day, faced with the task of handling a list of users, my objective was to filter out the inactive ones, magically transform them into Data Transfer Objects (DTOs), and subsequently compile them into a list. It was the quintessential scenario for leveraging Streams!
CopyList<UserDTO> activeUsers = users.stream()
.filter(User::isActive)
.map(UserDTO::new)
.collect(Collectors.toList());
Looks immaculate, doesn’t it? This solution functioned flawlessly during testing phases. Yet, it became clear in a production environment that we were grappling with significant performance headaches. An exhaustive debugging session soon unraveled the mystery.
The Pitfall That Lay in Ambush
Wherein lay the problem? The culprit was nestled within the UserDTO
constructor, which surreptitiously harbored a database call!
Copypublic UserDTO(User user) {
this.id = user.getId();
this.name = user.getName();
this.orders = orderService.getOrdersByUserId(user.getId()); // A glaring misstep!
}
Harnessing .map(UserDTO::new)
, I had unwittingly initiated N database calls—one per user—rather than a singularly efficient batch operation. Consequently, when managing lists with thousands of users, our database faced an onslaught, halting operations to a near standstill.
Implementing the Remedy
The remedy proved to be deceptively straightforward yet critical: initiate a batch query to retrieve all orders prior to mapping the users.
CopyMap<Long, List<Order>> ordersMap = orderService.getOrdersForUserIds(
users.stream().map(User::getId).collect(Collectors.toList())
);
List<UserDTO> activeUsers = users.stream()
.filter(User::isActive)
.map(user -> new UserDTO(user, ordersMap.get(user.getId())))
.collect(Collectors.toList());
Instead of a barrage of thousands of queries, this change distilled our operations into one streamlined batch query, greatly alleviating our database’s burden.
Insights and Takeaways
- While the power of Streams is undeniable, they aren’t a panacea for performance optimization.
- Beware of any concealed side effects lurking within
map()
orfilter()
. - Rigorously evaluate the database implications of your transformation operations.
The ordeal taught me a valuable lesson: complacency in understanding Java Streams led to this humbling yet enlightening episode. What about you? Have you ever stumbled upon a similarly elusive snare within Streams?