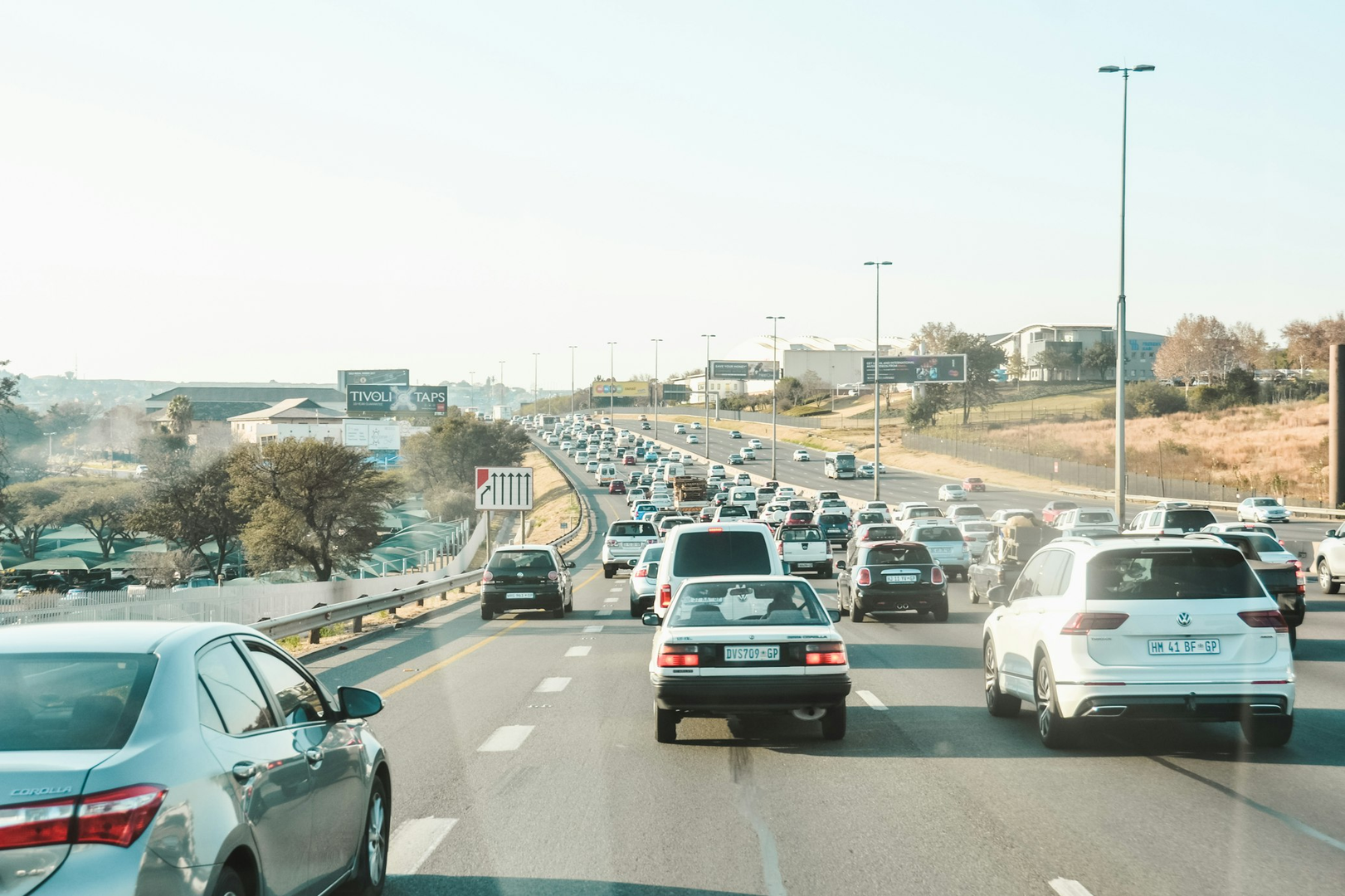
Node.js has rapidly ascended in popularity over the last decade, renowned for its prowess in managing concurrent connections and driving high-performance applications. Yet the pivotal query persists in the minds of countless developers: Is Node.js capable of supporting millions of users simultaneously?
The swift response is yes. However, the reality is layered and intricate. While Node.js is purposefully architected to scale, its performance under vast user loads is contingent upon your application’s architecture, the strategic optimizations you implement, and your adeptness in managing system resources.
In this article, we will delve into whether Node.js has the capacity to accommodate millions of users, what empowers its scalability, and the requisite steps to ensure that your Node.js application thrives under the duress of heavy traffic.
The Edge Node.js Holds in High-Traffic Environments
Fundamentally, Node.js is built upon an event-driven, non-blocking I/O paradigm. This design enables it to efficiently manage a multitude of concurrent connections. Traditional server constructs, such as those employing Apache or PHP, initiate new threads for each connection, taxing system resources substantially. In stark contrast, Node.js operates on a solitary thread, employing an event loop to process requests asynchronously, thereby not obligating one task to conclude before another begins.
Crucial Features That Enable Node.js to Scale:
- Non-blocking I/O: Manages multiple requests by commencing actions before others conclude.
- Event Loop: The nucleus of Node.js’s asynchronous functionality, which perpetuates efficient processing of incoming requests.
- V8 JavaScript Engine: Node.js relies on Google’s V8 engine, transforming JavaScript into highly optimized machine code, providing remarkable performance.
Though this framework is tailored for applications engaging in I/O-bound tasks, such as API servers, chat apps, and real-time services, scaling to accommodate millions of users transcends the innate features of Node.js. Let’s unpack the nuances of constructing a Node.js app that succeeds on this grand scale.
Navigating the Challenges of Scaling Node.js
Reaching millions of users involves more than just leveraging the underlying technology; it necessitates meticulous design and optimization of your application. When scaling Node.js, you might encounter significant hurdles, such as:
1. Limitations of the Single-Threaded Model
While the event-driven, single-threaded model is efficient, CPU-heavy tasks may obstruct the event loop, impairing your Node.js application’s overall performance. Applications performing intensive computations can lag since Node.js can’t attend to new requests during these operations.
Solution: Delegate CPU-intensive tasks to distinct worker threads or employ microservices to execute such operations separately.
const { Worker } = require('worker_threads');
const worker = new Worker('./heavyTask.js');
worker.on('message', result => {
console.log('Result from worker:', result);
});
2. Memory Leaks
As your Node.js application expands, poorly optimized code can induce memory leaks, particularly when handling extensive datasets or long-term applications. These leaks can increase memory demands, slowing down your server or provoking a crash amid heavy load.
Solution: Utilize tools like Chrome DevTools or the node — inspect flag to monitor memory usage and isolate leaks. Routinely inspect your app for areas where objects, variables, or event listeners may not be correctly released.
Strategies to Scale Node.js for Millions of Users
Scaling a Node.js application to support millions of users entails a balanced mix of strategic architectural practices, hardware augmentation, and resource management efficacy. Here’s how you achieve that:
1. Horizontal Scaling with Clusters
By default, Node.js operates on a single thread. However, you can leverage multi-core systems using the cluster module to run several instances of your application, each on a separate core. This allows your application to serve more users concurrently by distributing the workload across multiple processes.
Example:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World');
}).listen(8000);
}
In this scenario, the master process creates worker processes equal to the number of CPU cores available, letting each core handle requests and thereby enhancing overall throughput.
2. Load Balancing
Managing millions of users requires more than a single server. Load balancing distributes incoming traffic across multiple servers, ensuring that no one server is overburdened. Tools like NGINX, HAProxy, or cloud-based solutions such as AWS Elastic Load Balancer can help distribute your traffic across diverse Node.js instances.
3. Caching to Improve Performance
Fetching the same data from a database or executing API calls repetitively can be sluggish. Caching allows you to deliver commonly requested data quickly. Tools like Redis or Memcached can store data in memory, reducing database strain and enhancing response times.
Example:
const redis = require('redis');
const client = redis.createClient();
app.get('/data', async (req, res) => {
client.get('key', (err, data) => {
if (data) {
return res.send(JSON.parse(data));
} else {
const freshData = getFreshData(); // Fetch data from DB or API
client.set('key', JSON.stringify(freshData), 'EX', 3600); // Cache for 1 hour
return res.send(freshData);
}
});
});
4. Database Optimization
Database efficiency often becomes a constraint for many high-traffic applications. By refining database queries, implementing indexes, and limiting queries per request, you can noticeably boost application performance. As your user base expands, consider implementing database sharding or a read-replica architecture to distribute the load across several databases.
Showcasing Scalable Node.js Applications in the Real World
Several prominent companies utilize Node.js to power their high-traffic platforms, exemplifying that Node.js can indeed manage millions of users. Consider these examples:
- LinkedIn: The LinkedIn mobile server transitioned from Ruby on Rails to Node.js, experiencing a 20-fold reduction in servers while supporting over 600 million users.
- Netflix: Netflix employs Node.js to manage millions of simultaneous streams and cut down on startup times.
- Uber: Uber adopted Node.js for its highly scalable and real-time architecture, fundamental to managing their voluminous concurrent ride requests.
Concluding Thoughts: Scaling Node.js
Ultimately, can Node.js handle millions of users? Certainly—with the right architecture, optimizations, and scaling strategies in place. While the single-threaded model presents certain limitations, Node.js’s event-driven, non-blocking design aligns perfectly with I/O-bound tasks such as handling web traffic.
For those constructing a large-scale application with Node.js, it is crucial to implement horizontal scaling, utilize efficient load balancing, optimize databases, and employ caching. With these tactics, your Node.js application can adeptly manage millions of users without faltering.
Happy coding! 🚀