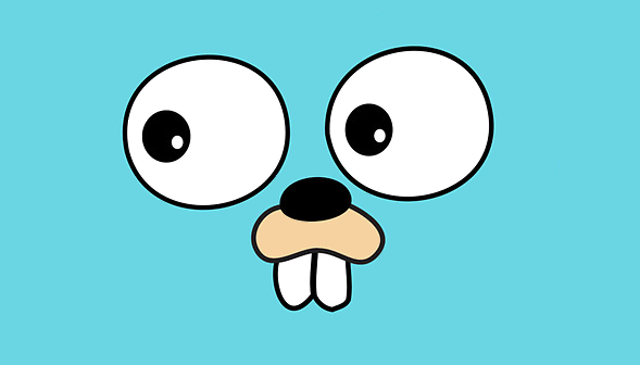
Stepping into a new organization focused on high-scale searches unveiled a crucial realization for me: design patterns are vital tools enabling us to conquer complexity effortlessly. Reflecting on my journey, I want to highlight five concurrency patterns in Go that could have been my saviors in both time and countless cups of coffee had I discovered them sooner. Join me on this exploration of efficiency!
Embracing the Worker Pool Pattern
Imagine staring at a formidable mountain of tasks, where launching a goroutine for each one is practically screaming inefficiency. Enter the dynamic Worker Pool pattern—your team of competent hands picking tasks one by one with precision. This approach not only optimizes concurrency by limiting the number of active goroutines but also adapts to the server’s processing capabilities.
Code Manifestation:
def worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d started job %d\n", id, j)
time.Sleep(time.Second) // Simulate work
results <- j * 2
fmt.Printf("Worker %d finished job %d\n", id, j)
}
}
func main() {
const numJobs = 5
jobs := make(chan int, numJobs)
results := make(chan int, numJobs)
// Start 3 workers
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
// Send jobs
for j := 1; j <= numJobs; j++ {
jobs <- j
}
close(jobs)
// Collect results
for a := 1; a <= numJobs; a++ {
<-results
}
}
Witness how seamlessly it handles concurrent requests, turning a daunting task into a manageable triumph.
Unveiling the Fan-Out, Fan-In Pattern
Visualize a river branching into streams, only to reconvene downstream. The Fan-Out, Fan-In pattern perfectly encapsulates this concept, distributing load across parallel goroutines and merging their results harmoniously.
Exemplifying Code:
def main() {
in := gen(2, 3, 4, 5)
// Fan-out
c1 := sq(in)
c2 := sq(in)
// Fan-in
for n := range merge(c1, c2) {
fmt.Println(n) // Outputs values like 4,9,16,25 in varying orders
}
}
func gen(nums ...int) chan int {
out := make(chan int)
go func() {
for _, n := range nums {
out <- n
}
close(out)
}()
return out
}
func sq(in chan int) chan int {
out := make(chan int)
go func() {
for n := range in {
out <- n * n
}
close(out)
}()
return out
}
func merge(cs ...chan int) chan int {
var wg sync.WaitGroup
out := make(chan int)
output := func(c chan int) {
for n := range c {
out <- n
}
wg.Done()
}
wg.Add(len(cs))
for _, c := range cs {
go output(c)
}
go func() {
wg.Wait()
close(out)
}()
return out
}
This pattern simplifies tasks such as web scraping, collecting data from diverse sources, and amalgamating them for further use.
Harnessing the Pipeline Pattern
Picture a meticulously organized factory assembly line, where each station enhances the product incrementally. The Pipeline pattern mirrors this production method, processing data step-by-step.
Implementing a Basic Pipeline:
def main() {
naturals := make(chan int)
squares := make(chan int)
// Counter
go func() {
for x := 0; x < 10; x++ {
naturals <- x
}
close(naturals)
}()
// Squarer
go func() {
for x := range naturals {
squares <- x * x
}
close(squares)
}()
// Printer
for x := range squares {
fmt.Println(x)
}
}
Perfect for streamlining data processing, this pattern showcases the magic of efficient transformation at each stage.
Utilizing Context for Graceful Cancellations
The art of managing goroutine lifecycles is indispensable, and the context
package stands as your trusted ally. Enable fluid cancellation of operations, which becomes a cornerstone in long-term applications.
A Practical Example:
def main() {
ctx, cancel := context.WithCancel(context.Background())
go func() {
// Emulate work
time.Sleep(2 * time.Second)
cancel() // Cancel context after 2 seconds
}()
select {
case <-time.After(5 * time.Second):
fmt.Println("Operation completed")
case <-ctx.Done():
fmt.Println("Operation canceled")
}
}
Notice how operations are canceled seamlessly after two seconds, safeguarding resources while fortifying application robustness.
Navigating Errors with the ErrGroup Pattern
Handling concurrent operation errors doesn’t have to be a conundrum. Enter the errgroup
package—a tool that elegantly centralizes errors from multiple goroutines, waiting for them to conclude before providing results.
Sample Implementation:
def main() {
var g errgroup.Group
urls := []string{
"https://golang.org",
"https://google.com",
"https://badhost", // This will induce an error
}
for _, url := range urls {
url := url // Prevent closure capture
g.Go(func() error {
resp, err := http.Get(url)
if err != nil {
return err
}
resp.Body.Close()
fmt.Println("Fetched:", url)
return nil
})
}
if err := g.Wait(); err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Successfully fetched all URLs")
}
}
Utilize errgroup
to streamline error collection, ideal for full-scale operations where every task’s success is crucial.
Concluding Thoughts
Concurrency in Go, though formidable, need not be an impenetrable labyrinth. These five patterns have reshaped my coding landscape:
- Worker Pool Pattern
- Fan-Out, Fan-In Pattern
- Pipeline Pattern
- Context for Cancellation
- ErrGroup Pattern
Initially challenging, their mastery reveals profound beauty and simplicity. If these insights resonated (or merely entertained), your feedback or reactions are warmly welcome. Keep coding with passion and efficiency! 🚀