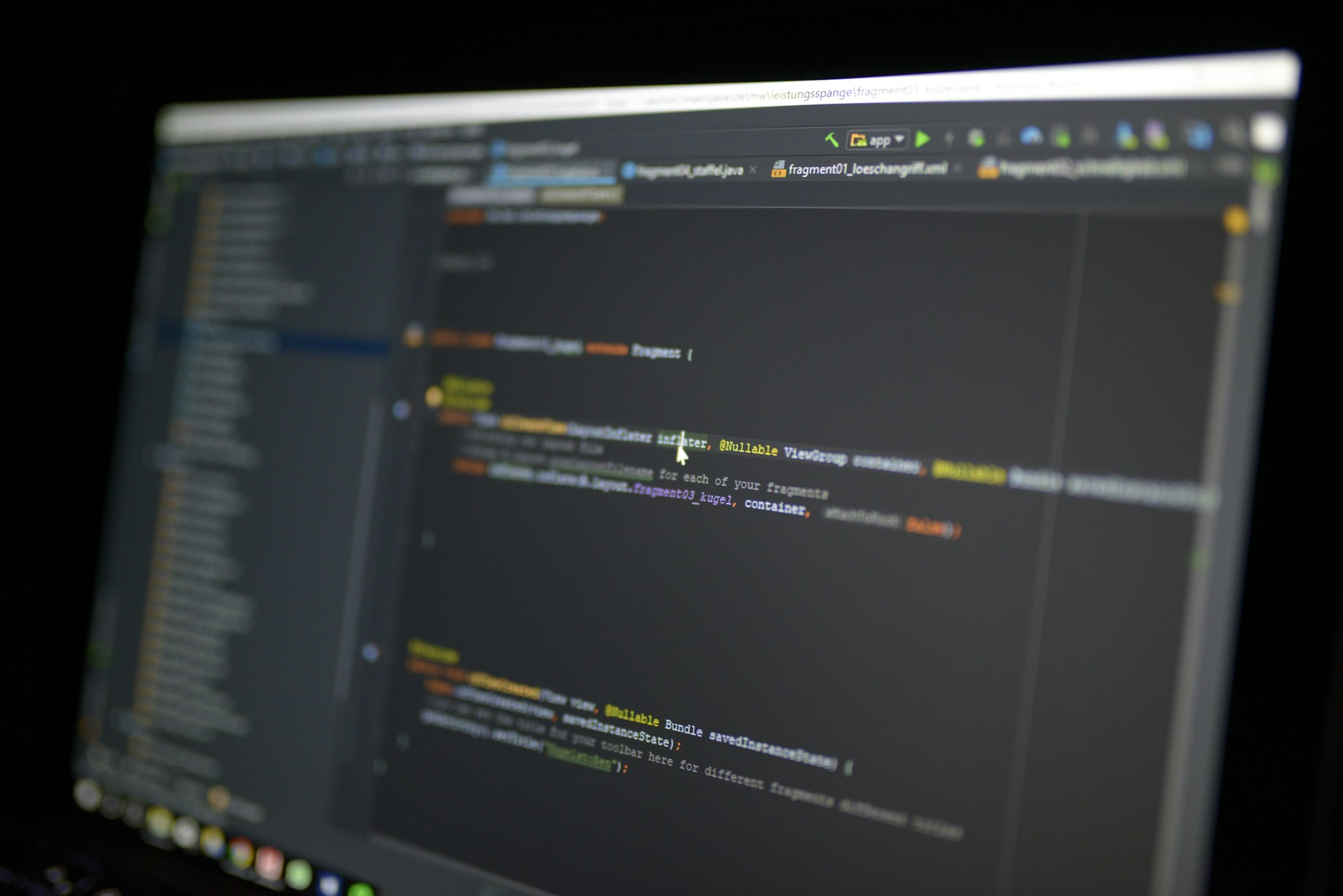
Spring Boot proudly stands as a beacon in the Java world, offering a robust framework for REST API development. Yet, as with any formidable tool, the path to mastery involves navigating through common pitfalls that can impair the elegance and efficiency of your code.
Below, we explore seven frequent missteps encountered in Spring Boot development and offer strategies to sidestep them effectively.
Mastering HTTP Methods
A critical aspect of crafting APIs is selecting the correct HTTP methods to maintain clarity and consistency.
Less Effective Implementations:
@PostMapping("/users/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
return userService.updateUser(id, user);
}
@GetMapping("/users/create")
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
Optimized Approaches:
@PutMapping("/users/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
return userService.updateUser(id, user);
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
Proper HTTP methods to consider:
- GET: Retrieve data
- POST: Create new resources
- PUT: Update existing resources
- DELETE: Remove resources
- PATCH: Partial updates
Exceptional Exception Handling
Handling exceptions with finesse prevents chaos, delivers clear error messaging, and seals potential security gaps.
Flawed Technique:
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
try {
return userService.getUser(id);
} catch (Exception e) {
return null; // Not ideal
}
}
Improving Technique:
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<ErrorResponse> handleUserNotFoundException(UserNotFoundException ex) {
ErrorResponse error = new ErrorResponse(
HttpStatus.NOT_FOUND.value(),
ex.getMessage(),
LocalDateTime.now()
);
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(ValidationException.class)
public ResponseEntity<ErrorResponse> handleValidationException(ValidationException ex) {
ErrorResponse error = new ErrorResponse(
HttpStatus.BAD_REQUEST.value(),
ex.getMessage(),
LocalDateTime.now()
);
return new ResponseEntity<>(error, HttpStatus.BAD_REQUEST);
}
}
@Getter
@AllArgsConstructor
public class ErrorResponse {
private int status;
private String message;
private LocalDateTime timestamp;
}
Vigilant Input Validation
Neglecting input validation can lead to data integrity issues and security vulnerabilities.
Unsound Setup:
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
public class User {
private String email;
private String password;
private String phoneNumber;
}
Sound Setup:
@PostMapping("/users")
public User createUser(@Valid @RequestBody User user) {
return userService.createUser(user);
}
public class User {
@Email(message = "Invalid email format")
@NotNull(message = "Email is required")
private String email;
@Size(min = 8, message = "Password must be at least 8 characters long")
@Pattern(regexp = "^(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=]).*$",
message = "Password must contain at least one digit, one uppercase, one lowercase, and one special character")
private String password;
@Pattern(regexp = "^\\+?[1-9]\\d{1,14}$", message = "Invalid phone number format")
private String phoneNumber;
}
Establishing Naming Clarity
Inconsistent naming conventions obscure code comprehension, reducing usability.
Lack of Clarity:
@RestController
public class UserController {
@GetMapping("/getUsers")
public List<User> getUsers() { ... }
@PostMapping("/createNewUser")
public User createNewUser(@RequestBody User user) { ... }
@PutMapping("/updateUserDetails/{userId}")
public User updateUserDetails(@PathVariable Long userId) { ... }
}
Improved Structure:
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
@GetMapping
public List<User> getUsers() { ... }
@PostMapping
public User createUser(@RequestBody User user) { ... }
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id) { ... }
}
Navigating Data Deluge with Pagination
Ignoring pagination strains performance, degrading user experience.
Unsustainable Approach:
@GetMapping("/users")
public List<User> getAllUsers() {
return userRepository.findAll(); // May return an unwieldy dataset
}
Sustainable Solution:
@GetMapping("/users")
public Page<User> getUsers(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "20") int size,
@RequestParam(defaultValue = "id") String sortBy
) {
Pageable pageable = PageRequest.of(page, size, Sort.by(sortBy));
return userRepository.findAll(pageable);
}
// Repository
public interface UserRepository extends PagingAndSortingRepository<User, Long> {
Page<User> findByLastName(String lastName, Pageable pageable);
}
Guarding Against Data Exposure
Safeguarding sensitive information during serialization and logging is crucial for security.
Risky Release:
@Entity
public class User {
private Long id;
private String username;
private String password; // Vulnerable to exposure
private String ssn; // Vulnerable to exposure
// Getters and setters
}
Secure Management:
@Entity
public class User {
private Long id;
private String username;
@JsonIgnore
private String password;
@JsonIgnore
private String ssn;
// Getters and setters
}
// Use DTOs for responses
@Data
public class UserDTO {
private Long id;
private String username;
private LocalDateTime createdAt;
public static UserDTO fromEntity(User user) {
UserDTO dto = new UserDTO();
dto.setId(user.getId());
dto.setUsername(user.getUsername());
dto.setCreatedAt(user.getCreatedAt());
return dto;
}
}
Perfecting Response Status Codes
Mismatched response status codes cloud API usability, creating confusion.
Misaligned Method:
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userService.createUser(user); // Incorrect status code
}
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
User user = userService.findById(id);
if (user == null) {
return new User(); // Returns empty object instead of 404
}
return user;
}
Corrective Method:
@PostMapping("/users")
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.createUser(user);
return new ResponseEntity<>(createdUser, HttpStatus.CREATED);
}
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
return userService.findById(id)
.map(user -> ResponseEntity.ok(user))
.orElse(ResponseEntity.notFound().build());
}