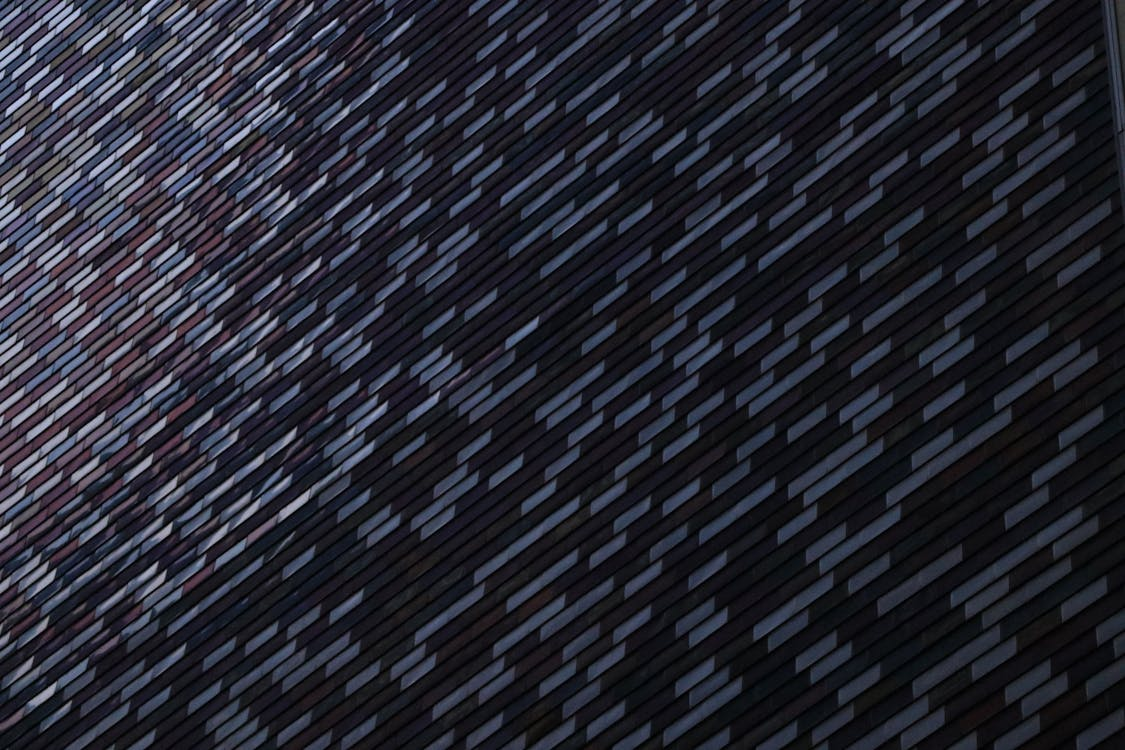
Microservices are transforming the landscape of software development. By deconstructing massive applications into smaller, autonomous services, they champion a more agile and scalable system architecture. Yet, this newfound adaptability introduces its share of complexity. Enter design patternsâtime-tested blueprints that help mitigate common challenges.
Here’s a journey into five crucial microservices design patterns poised to lead development in 2025, elucidated through straightforward examples.
1. API Gateway Pattern
Picture yourself browsing an online store. The website connects with diverse backend servicesâhandling user profiles, orders, and payments. Directly linking your application to all these services can slow it down and complicate maintenance. This is where the API Gateway takes center stage.
Solution:
The API Gateway operates as a unified entry-point, receiving client requests and directing them to the suitable backend services.
How It Works:
- The client issues a request to the API Gateway.
- The Gateway routes this request to the aligned service(s).
- It can consolidate responses if necessary and relay them back to the client.
Example: Using Spring Cloud Gateway
Copy@EnableGateway
@SpringBootApplication
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("user-service", r -> r.path("/users/**")
.uri("lb://USER-SERVICE"))
.route("order-service", r -> r.path("/orders/**")
.uri("lb://ORDER-SERVICE"))
.build();
}
}
This configuration streamlines interaction with various services through a singular gateway.
Best Practices:
- Enhance with caching to mitigate redundant requests.
- Fortify with security protocols like OAuth2 or JWT.
- Manage service failures using circuit breakers (explored below).
2. Saga Pattern
Engaging multiple services within a transaction complicates the process. Consider the scenario of placing an orderâone service deducts the payment, another updates inventory, and a third finalizes the order. The Saga Pattern ensures that all actions are synchronized, or everything is efficiently reversed in the event of a failure.
Solution:
Saga breaks a transaction into orchestrated steps, with each service managing its role and invoking the subsequent action. In event of failure, services can roll back.
Types of Sagas:
- Choreography: Services autonomously determine their next move.
- Orchestration: A central conductor coordinates the flow.
Example: Choreography-Based Saga
- Credit is reserved by the user service.
- The order service logs the order.
- The payment service finalizes the transaction.
Code Snippet:
Copy@EventListener
public void handleOrderCreatedEvent(OrderCreatedEvent event) {
PaymentRequest paymentRequest = new PaymentRequest(event.getOrderId(), event.getAmount());
paymentService.processPayment(paymentRequest);
}
Best Practices:
- Design steps to handle retrials gracefully.
- Maintain logs to diagnose any flaws.
- Rigorously test sagas to prevent data discrepancies.
3. Circuit Breaker Pattern
A failing service can ripple through a system, causing widespread issuesâsuch as a downed payment service halting all orders. The Circuit Breaker Pattern serves as a safeguard by pausing requests to a struggling service until recovery.
Solution:
A circuit breaker toggles effectively between states:
- Closed State: Normal request flow.
- Open State: Suspends requests to shield system integrity.
- Half-Open State: Sends limited requests to test service functionality.
Example: Using Resilience4j
Copy@CircuitBreaker(name = "userService", fallbackMethod = "fallbackGetUser")
public User getUser(String userId) {
return webClient.get()
.uri("http://user-service/users/" + userId)
.retrieve()
.bodyToMono(User.class)
.block();
}
public User fallbackGetUser(String userId, Throwable throwable) {
return new User("default", "Guest");
}
When the user service is unavailable, the fallback offers a default user.
Best Practices:
- Pair with retries for ephemeral malfunctions.
- Analyze metrics to adjust parameters.
- Deliver significant fallbacks to prevent user frustration.
4. Event Sourcing Pattern
Traditional systems capture only the current state of data, like an account balance. But if you need a chronological account of changesâevent sourcing is the answer, logging each modification as an event.
Solution:
Transformations are preserved as events within a store, allowing for historical data reconstruction.
Example: Order Service Event Sourcing
Copypublic class OrderCreatedEvent {
private String orderId;
private String userId;
private List<String> items;
// Getters and Setters
}
// Storing an event
EventStore.save(new OrderCreatedEvent(orderId, userId, items));
Best Practices:
- Utilize platforms like Apache Kafka for event management.
- Periodically capture snapshots to accelerate state reassembly.
- Ensure events are immutable and thoroughly documented.
5. Strangler Fig Pattern
Modernizing daunting monolithic systems can be an arduous task. The Strangler Fig Pattern elegantly allows gradual migration to microservices, minimizing risk by incrementally phasing out legacy system segments.
Solution:
Incrementally replace the old architecture with microservices, allowing the legacy system to gradually recede.
Example:
- Identify the monolith feature to phase out (e.g., user management).
- Introduce a microservice for the new functionality.
- Redirect
/users
requests via an API Gateway to the fresh service.
Gateway Configuration:
Copyroutes:
- id: user-service
uri: lb://USER-SERVICE
predicates:
- Path=/users/**
Best Practices:
- Commence with lower-risk features.
- Use metrics for performance and issue monitoring during transition.
- Keep detailed records of all alterations throughout the migration.
Conclusion
Embrace the depth and dexterity of these design patterns to enhance your projectâs resilience in the face of future uncertainties. How have these patterns shaped your projects? Share in the comments below and letâs build more robust systems together!