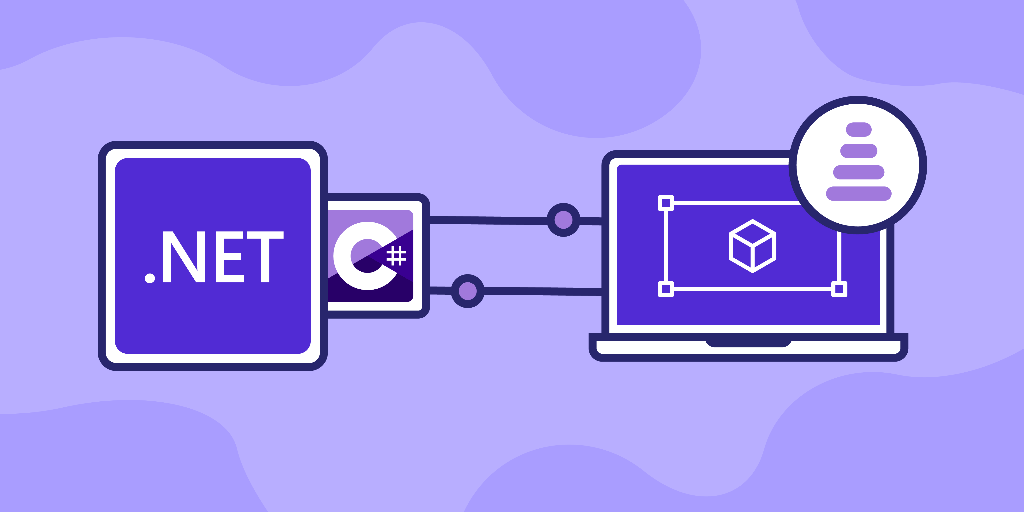
Unveiling the Path to .NET Excellence
Whether you’re new to the world of development or have a couple of years under your belt, these insights arise from authentic, real-life experiencesâmistakes humbled, lessons crystallized, and wisdom earned.
This guide compiles invaluable tips and tricks amassed over years of dedication.
1. Conquer Asynchronous Programming
At the dawn of my journey with .NET, the rise of async/await was apparent. My habitual synchronous API calls frequently crumbled under stress. Embracing async patterns in C# transformed my approach radically. Engage Task.Run
prudently, steer clear of async void
, and always opt for ConfigureAwait(false)
when crafting library code.
Example:
public async Task<string> FetchDataAsync(HttpClient client)
{
var response = await client.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
2. Dependency Injection: A Fundamental Paradigm
On one occasion, I handled a legacy .NET Framework project laden with hard-coded dependencies. The shift to Dependency Injection (DI) was a challenging but enlightening revelation. DI ensures your code is testable and modular, setting the groundwork for robust applications.
Example:
public interface IDataService
{
string GetData();
}
public class DataService : IDataService
{
public string GetData() => "Hello, Dependency Injection!";
}
Integrate it into your DI container:
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddScoped<IDataService, DataService>();
3. Embrace Records and Immutable Structures
The introduction of records in C# 9 revolutionized unnecessary boilerplate. Use records for immutable data structures whenever possible.
Example:
public record Person(string Name, int Age);
This automatically provisions equality checks and immutability.
4. Exploit Pattern Matching
Pattern matching in C# is transformative. I’ve witnessed codebases laden with cumbersome if-else
chains that could have been rejuvenated with pattern matching.
Example:
static string GetMessage(object obj) => obj switch
{
int number => $"Number: {number}",
string text => $"Text: {text}",
_ => "Unknown type"
};
5. Cautious Use of Reflection
The allure of reflection is undeniable, yet it exacts a performance toll. Early in my career, I overrelied on dynamic method invocations, only to lament it later. Opt for generics or interfaces for increased type safety and performance improvements.
Bad Example:
var method = typeof(MyClass).GetMethod("MyMethod");
method.Invoke(instance, null);
6. Optimize Your LINQ Queries
While LINQ’s elegance is unmatched, recklessness can degrade performance. Be conscious of deferred executions and prevent redundant iterations.
Example:
var result = myCollection.Where(x => x.IsActive).Select(x => x.Name).ToList();
ToList()
should be invoked only when imperative to avoid multifold executions.
7. Choose String Interpolation Over Concatenation
String interpolation offers readability and execution efficiency.
Bad:
string message = "Hello " + name + "!";
Good:
string message = $"Hello {name}!";
8. Exception Handling with Precision
Catching generic exceptions is a rookie misstep. Handle specific ones and dodge squashing them.
Bad:
try { /* Code */ }
catch (Exception) { /* Do nothing */ }
Good:
try { /* Code */ }
catch (IOException ex) { Log(ex.Message); }
9. Resist Premature Optimization
One of my seminal errors as a junior was premature optimization. Always profile before proceeding with optimizations.
10. Harness Span<T>
and Memory<T>
for Peak Performance
The advent of Span<T>
and Memory<T>
in .NET Core dramatically boosted performance for managing extensive data sets.
Example:
Span<int> numbers = stackalloc int[] { 1, 2, 3, 4 };
11. Log Intelligently
While insufficient logging misguides, excessiveness can inundate logs. Employ structured logging with tools like Serilog or NLog.
12. Fortify Your .NET Applications
Utilize IOptions<T>
for housing sensitive configuration values rather than embedding secrets within your codebase.
13. Explore .NET Performance Profiling Tools
Tools like dotTrace and BenchmarkDotNet are indispensable for measuring and enhancing performance.
14. Unit Testing is Non-Negotiable
Any seasoned developer dreads the disruption caused by a breaking change. Eternally write unit tests using frameworks such as xUnit or NUnit.
Example:
[Fact]
public void Add_ShouldReturnSum()
{
int result = Add(2, 3);
Assert.Equal(5, result);
}
15. Employ Source Generators
With C# 10, source generators amplify your capacity to automate repetitive code production during compile time.
16. Streamline with Minimal APIs in .NET 6+
Minimal APIs trim boilerplate and ease development.
Example:
var app = WebApplication.Create();
app.MapGet("/hello", () => "Hello World");
app.Run();
17. An Infinite Cycle of Learning and Sharing
The most accomplished developers perpetually learn and propagate knowledge.
Conclusion
Being a senior .NET developer encompasses more than merely crafting code. It entails an ongoing commitment to producing applications that are maintainable, efficient, and secure. Take these lessons to heart and pave the way for both personal and communal advancement.