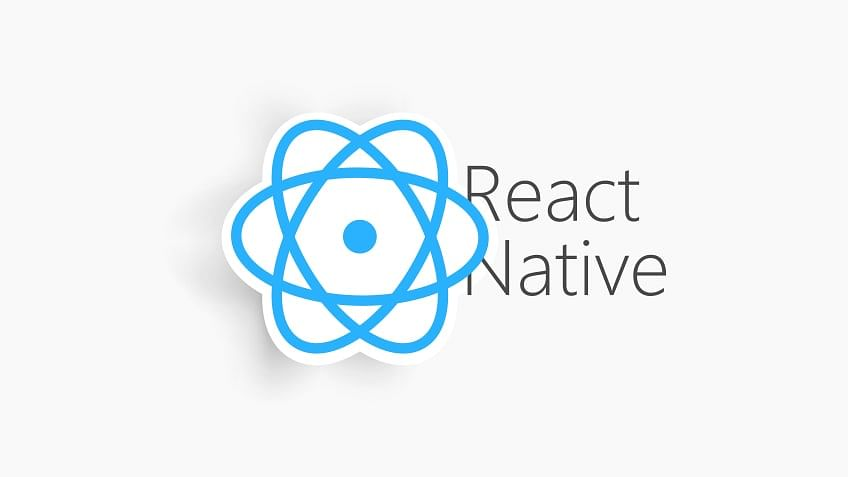
Greetings, fellow developers! Over the past three years, I’ve journeyed deep into the world of React Native, crafting apps that either soared to success or crashed with the grandeur of an unhandled JavaScript promise. Through these projects, I’ve encountered missteps, tackled performance challenges, and absorbed hard-earned lessons that go beyond what any tutorial could teach. Whether you’re just dipping your toes into React Native or questioning your life decisions, let’s explore some pearls of wisdom I wish someone had emphasized in Comic Sans bold.
1. Beyond Global State: Understanding Context and Its Limitations
In my inaugural app, I wielded React’s Context API like an indiscriminate adhesive. Whether it was managing authentication, toggling themes, or even counting values, Context became my go-to solution. By the time I developed my third app, this approach had mutated into a dependency nightmare that reacted unpredictably to any insignificant change.
Key Insights:
- Distinguish server state from global state: Embrace tools like React Query or SWR for superior data handling, caching, and synchronization instead of cramming everything into Redux or Context. Reserve global state for truly universal needs, such as user preferences.
- Opt for Zustand in smaller projects: Its API is more streamlined than Redux, offering efficient state management without unnecessary re-renders.
// Zustand example: A clean, minimal store
import create from 'zustand';
const useCartStore = create((set) => ({
items: [],
addItem: (item) => set((state) => ({ items: [...state.items, item] })),
}));
2. The Navigational Challenge: Steering Through Complexity
React Navigation can feel like a blessing until it suddenly turns into a labyrinth. Initially, I structured my navigation containers with little foresight, only to later find myself entangled in hours of debugging as goBack()
behaved unpredictably.
Key Insights:
- Design your navigation architecture early: Establish a root
NavigationContainer
and adhere to a coherent hierarchy (e.g., Stack -> Tabs -> Screens). - Implement type-checking for navigators: Employ TypeScript or PropTypes to prevent invalid parameters. “Undefined is not an object” errors will otherwise become a recurring nightmare.
- Incorporate deep linking from the outset: Your project manager will inevitably request it post-launch, and preparation will save you headaches.
3. Native Modules Demystified: Fear Not the Language Barrier
For the longest time, I treated native code like a relic from a bygone era. That illusion shattered when I had to integrate a niche SDK, and the comfort of “expo install” was no longer sufficient.
Key Insights:
- Familiarize yourself with native modules early on: You don’t need to master Swift or Java, but a basic understanding of bridging native code is invaluable.
- Leverage
react-native-builder-bob
: It streamlines the creation of native modules, allowing you to concentrate on functionality rather than grappling with Xcode tantrums. - Embrace Expo Modules API: If you’re using Expo, their modules system simplifies native integration.
4. Performance as a Non-Negotiable Feature
My first app’s performance was sluggish, akin to a never-ending Windows update, because I imprudently utilized ScrollView
for a massive 1,000-item list. Spoiler alert: the app didn’t just crash; my self-confidence took a hit too.
Key Insights:
- Prefer
FlatList
andSectionList
: These components efficiently recycle visual elements, much like a thrift store. UtilizegetItemLayout
for fixed-height items to avoid costly measurements. - Memoize judiciously: Enclose components in
React.memo
and deployuseCallback
for functions passed to child components. However, avoid excessive memoization; it’s a spice, not the main ingredient. - Profile performance with Flipper: This invaluable React Native tool allows you to scrutinize re-renders, network activity, and native logs, offering an X-ray vision into your appās performance.
5. Avoid Over-Engineering: Simplify Your Approach
I once devised an intricately “scalable” folder structure with ten levels of nesting for a simple to-do app. My team member still mocks me for it at gatherings.
Key Insights:
- Begin with Expo: Unless custom native code is imperative, Expo accelerates your MVP development. Eject later if absolutely necessary.
- Favor straightforward code over cunning solutions: That slick animated gradient button is likely to malfunction come Android 14. Instead, rely on community libraries (but always review their GitHub issues first!).
- Don’t shun copy-pasting: Itās fine to duplicate solutions across different areas unless itās the same problem thriceāthen encapsulate it.
Reflections: Embrace the Journey
React Native is a dynamic rollercoasterāthrilling, occasionally overwhelming, and unpredictably fiery. Accepting that no one achieves perfection on their first attempt accelerates your journey to developing apps that won’t become meme material on Twitter.
Have you uncovered any React Native revelations on your path? Let’s share experiences and perhaps a tear or two in the comments below. š