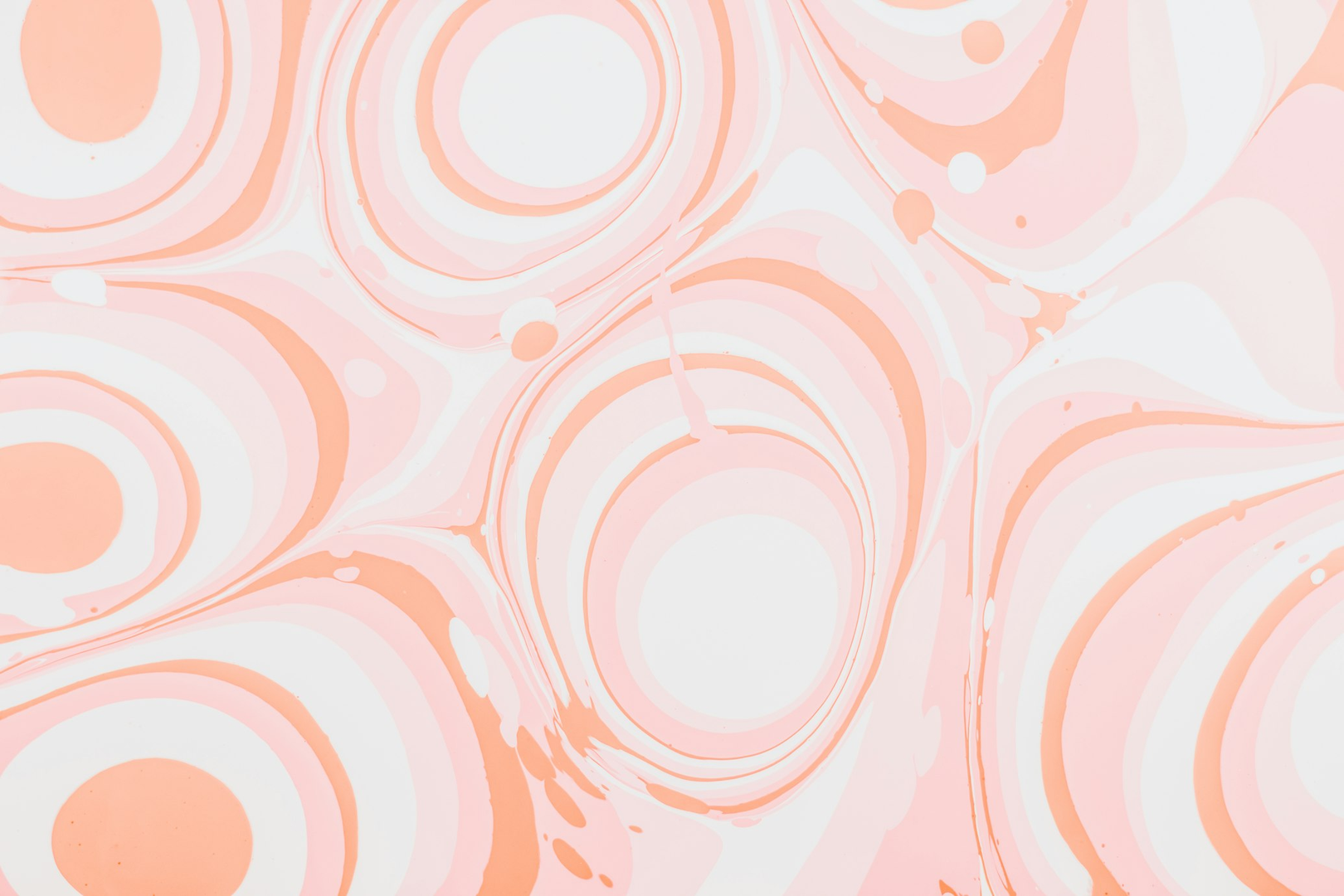
In the ever-evolving landscape of software development, microservices have ushered in a revolution. This transformative approach dissects the traditional monolith into finely-tuned, independent services, each excelling in its singular function. The allure lies in its promise of scalability and flexibility, but these benefits come intertwined with new layers of complexity. To navigate this intricate web, design patterns serve as our guideāproven strategies that offer clarity and direction.
As we stride into 2025, familiarize yourself with these five pivotal microservices design patterns, demystified through practical scenarios.
1. API Gateway Pattern: The Storefront to Your Services
Consider your experience as an online shopper. Behind the scenes, the platform interacts with multiple backend servicesāmanaging users, processing orders, and handling payments. Direct connections would be chaotic and cumbersome. Enter the API Gateway.
Strategic Resolution:
The API Gateway streamlines communication, acting as a singular conduit. Clients send one unified request, and the gateway efficiently channels it to the appropriate backend services.
Operational Overview:
- The client submits a request to the API Gateway.
- The gateway assigns the request to the pertinent service(s).
- Optionally, it consolidates responses, sending cohesive data back to the client.
Illustration: Implementing Spring Cloud Gateway
@EnableGateway
@SpringBootApplication
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("user-service", r -> r.path("/users/**")
.uri("lb://USER-SERVICE"))
.route("order-service", r -> r.path("/orders/**")
.uri("lb://ORDER-SERVICE"))
.build();
}
}
This configuration creates a seamless interface for clients to engage with multiple services via a unified gateway.
Optimal Practices:
- Integrate caching to alleviate repetitive data requests.
- Fortify with security measures like OAuth2 or JWT.
- Employ circuit breakers for handling service disruptions effectively.
2. Saga Pattern: Orchestrating Multi-Service Transactions
Complex transactions spanning multiple services pose unique challenges. Imagine ordering a product: money must be deducted, inventory updated, and the order created. If one piece fails, the entire sequence is jeopardized. The Saga Pattern ensures transactional integrity and compensatory actions.
Strategic Resolution:
Segment transactions into smaller, manageable steps. Each service executes its operation and signals the next, rolling back if errors arise.
Variations of Sagas:
- Choreography: Each service autonomously determines subsequent actions.
- Orchestration: Centralized control orchestrates the entire process.
Illustration: Choreography-Driven Saga
- Reserve credit in the user service.
- Proceed to order creation.
- Finalize payment operations.
Code Insight:
@EventListener
public void handleOrderCreatedEvent(OrderCreatedEvent event) {
PaymentRequest paymentRequest = new PaymentRequest(event.getOrderId(), event.getAmount());
paymentService.processPayment(paymentRequest);
}
Optimal Practices:
- Design steps for seamless retries without errors.
- Maintain comprehensive logs for transaction tracking.
- Conduct thorough testing to preempt data inconsistencies.
3. Circuit Breaker Pattern: Protecting System Stability
System failures and sluggish services can ripple across an infrastructure, potentially crippling operations. If a payment service falters, all related transactions might collapse. The Circuit Breaker Pattern offers a safeguard, pausing requests to failing services for recovery.
Strategic Resolution:
The circuit breaker functions as a strategic switch:
- Closed State: Regular request flow.
- Open State: Blocks requests to guard system stability.
- Half-Open State: Sends selective requests, probing service recovery.
Illustration: Applying Resilience4j
@CircuitBreaker(name = "userService", fallbackMethod = "fallbackGetUser")
public User getUser(String userId) {
return webClient.get()
.uri("http://user-service/users/" + userId)
.retrieve()
.bodyToMono(User.class)
.block();
}
public User fallbackGetUser(String userId, Throwable throwable) {
return new User("default", "Guest");
}
When the service is unreachable, a predefined fallback response mitigates impact.
Optimal Practices:
- Couple with retry mechanisms to handle transient errors.
- Track performance metrics to fine-tune thresholds.
- Offer substantial fallbacks to maintain user experience.
4. Event Sourcing Pattern: Chronicling Data Evolution
Traditional systems often only capture the present state, like an account balance. But when past changes are crucial, event sourcing records each state transition as an event, preserving a comprehensive historical record.
Strategic Resolution:
Instead of overwriting data, record each state change as an event within an event store. Replay these events to reconstruct the timeline of changes.
Illustration: Order Service with Event Sourcing
public class OrderCreatedEvent {
private String orderId;
private String userId;
private List<String> items;
// Getters and Setters
}
// Archiving an event
EventStore.save(new OrderCreatedEvent(orderId, userId, items));
Optimal Practices:
- Utilize platforms like Apache Kafka for event storage.
- Implement snapshots to expedite current state recovery.
- Ensure events are immutable and well-documented for historical accuracy.
5. Strangler Fig Pattern: Evolving Systems Incrementally
Many enterprises are tethered to aging, cumbersome systems. An immediate overhaul is fraught with risk. The Strangler Fig Pattern enables a phased transition, ushering modernization incrementally.
Strategic Resolution:
Gradually supplant segments of the legacy system with microservices, allowing the old to diminish as new replaces it.
Illustration:
- Designate an aspect of the monolith (e.g., user management) for replacement.
- Implement a microservice to provision new functionality.
- Deploy an API Gateway to redirect requests to the new service.
Gateway Configuration:
routes:
- id: user-service
uri: lb://USER-SERVICE
predicates:
- Path=/users/**
Optimal Practices:
- Begin with smaller, lower-risk features for transition.
- Monitor metrics vigilantly for performance and migration-related issues.
- Maintain meticulous documentation to facilitate tracking of migration progress.
Envisioning the Future
Embrace the learning and integration of these patterns within your projects. They are the blueprints for crafting systems poised for imminent and future technological challenges.
Have you implemented any of these patterns? Share your insights and experiences in the comments to foster a collaborative learning environment!