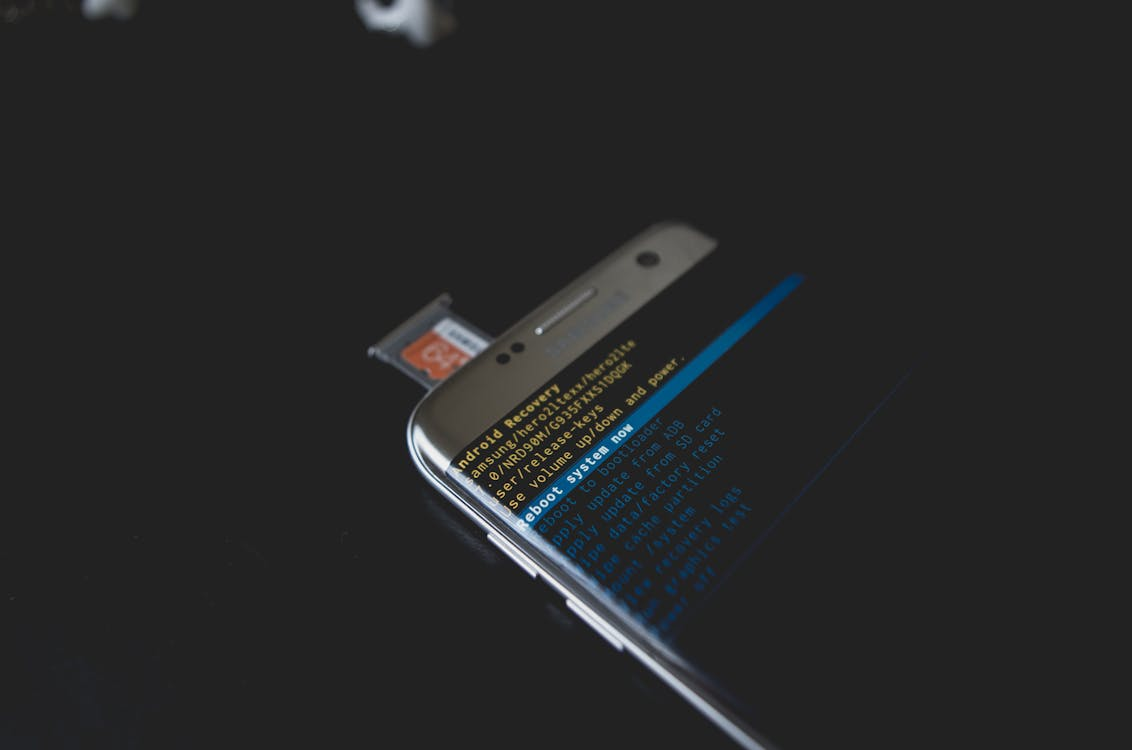
Android 14 has transformed the landscape of foreground services, stipulating changes vital for apps targeting SDK version 34. Let’s delve into these modifications and what developers must do to align.
Target Deadline: As of August 31, 2024, new app releases and updates must target SDK version 34+.
We’ll also address common exceptions and their resolutions, culminating in a sample project exemplifying correct foreground service implementation.
Understanding Foreground Services
Foreground services are your app’s workhorses, performing visible operations that continue even with the app in the background. These services necessitate displaying a system notification to inform users of their active status and resource utilization.
Consider these applications:
- A music player, like Spotify, streaming tunes regardless of app interaction.
- A fitness tracker, such as Google Fit, monitoring steps on a locked device.
- Navigation tools like Google Maps, providing seamless directions.
Foreground Service Categories
The evolution of foreground services took a leap with Android 10, introducing the android:foregroundServiceType
attribute in the <service>
element. Initially crucial for services involving location, camera, or microphone permissions, it now mandates type declaration in Android 14, ensuring consistent usage across devices.
Here are the available service types:
camera
β For apps accessing the camera from the background (video calls, etc.)connectedDevice
β For interactions with external devices, e.g., Bluetooth fitness gadgetsdataSync
β Note: deprecated; opt for alternatives likeDownloadManager
health
(new) β Health-related functions, such as exercise monitoringlocation
β Essential for location-based services like navigationmediaPlayback
β For background audio or video streamingmediaProjection
β Projects content to external displaysmicrophone
β For apps using the background microphonephoneCall
β Facilitates ongoing callsremoteMessaging
(new) β Transfers messages across devicesshortService
β Executes essential tasks swiftly, capped at around 3 minutesspecialUse
β Covers unique use casessystemExempted
β Reserved for system-level apps
Declaration of Foreground Service Type
Updating your AndroidManifest
file to specify the appropriate service type is fundamental for Android 14 compatibility.
If your service involves various categories, combine them using the |
operator:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" ...>
<service
android:name=".MyForegroundService"
android:foregroundServiceType="camera|location|microphone"
android:exported="false">
</service>
</manifest>
Initiating a foreground service sans type declaration results in a MissingForegroundServiceTypeException
upon startForeground()
invocation.
Securing Specific Foreground Service Permissions
From Android 9 onwards (API 28
), applications must declare FOREGROUND_SERVICE
in their manifest, automatically granted by the system.
<uses-permission android:name="android.permission.FOREGROUND_SERVICE"/>
Android 14 elevates this by necessitating service-type-specific permissions. For instance, interfacing with a Bluetooth device mandates FOREGROUND_SERVICE_CONNECTED_DEVICE
. These permissions remain system-granted.
<uses-permission android:name="android.permission.FOREGROUND_SERVICE_CONNECTED_DEVICE"/>
Overlooking these permissions results in a SecurityException
, detailing the oversight:
java.lang.SecurityException:
Permission Denial: startForeground from pid=8589, uid=10623
requires android.permission.FOREGROUND_SERVICE
or
java.lang.SecurityException:
Starting FGS with type mediaPlayback targetSDK=34
requires permissions:
all of the permissions allOf=true
[android.permission.FOREGROUND_SERVICE_MEDIA_PLAYBACK]
Calling startForeground
with Precision
Beyond manifest declarations, specifying service types during startForeground()
invocation is imperative.
To activate the foreground service, integrate ServiceCompat.startForeground()
into onStartCommand()
. This method demands the service instance, notification ID, notification object, and service types.
Prior techniques involved passing 0
for foregroundServiceType
, now obsolete in favor of explicit type entry or subsets of manifest declarations. It’s viable to invoke startForeground()
subsequently for additional types.
ServiceCompat.startForeground(
this,
id,
notification,
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R) {
ServiceInfo.FOREGROUND_SERVICE_TYPE_MEDIA_PLAYBACK
} else {
0
}
)
Passing 0
or undeclared types triggers exceptions:
-
android.app.InvalidForegroundServiceTypeException: Starting FGS with type none targetSDK=34 has been prohibited
-
java.lang.IllegalArgumentException: foregroundServiceType 0x00000002 is not a subset of foregroundServiceType attribute 0x00000000 in service element of manifest file
Notably,
startForeground()
must be executed within 10 seconds postonStartCommand()
to sidestepandroid.app.ForegroundServiceDidNotStartInTimeException
.
Ensuring Runtime Permissions
Each foreground service type entails distinct permissions. Secure these runtime permissions prior to service initiation to avert exceptions. For instance, background camera usage necessitates android.permission.CAMERA
.
Bluetooth device connections require satisfying at least one of these conditions:
- Manifest permissions:
CHANGE_NETWORK_STATE
,CHANGE_WIFI_STATE
,CHANGE_WIFI_MULTICAST_STATE
,NFC
,TRANSMIT_IR
- Runtime permissions:
BLUETOOTH_CONNECT
,BLUETOOTH_ADVERTISE
,BLUETOOTH_SCAN
,UWB_RANGING
- Invoke
UsbManager.requestPermission()
Specific requirements for all types are detailed in the official documentation.
Failing to meet these conditions results in an informative exception:
Starting FGS with type connectedDevice targetSDK=34 requires permissions:
- all of the permissions allOf=true
- [android.permission.FOREGROUND_SERVICE_CONNECTED_DEVICE]
- any of the permissions allOf=false
- [android.permission.BLUETOOTH_ADVERTISE, ...]
Proper Notification Configuration
Foreground services demand a visible notification throughout execution. Since Android 13, notifications necessitate runtime permission, granted by user consent.
- Post requesting
POST_NOTIFICATIONS
:- Consent granted = Visible notification.
- Consent denied = Task manager reflects background activity.
- No request = Same as denial scenario.
Google Play Console Submissions
Submitting an app targeting Android 14 on the Play Console requires details regarding your foreground service use. This transparency aids in verifying appropriate service deployment.
Your declaration will include:
- Explanation of app functionalities utilizing such services
- Impact of system interruption or deferment
- A demo video displaying each service functionality
- Selection of predefined use cases or custom inputs from here
Note:
shortService
andsystemExempted
are exempt from this declaration.
Refer to this official support page for comprehensive instructions and updates.
Enhancements on Samsung Devices
Samsung, working alongside Google, has standardized foreground service policies for Galaxy devices on Android 14+. Given Samsung’s dominance (34% market share), this promises a cohesive Android platform, aligning closely with Google’s policies.
“This partnership with Google towards a unified policy assures a consistent, reliable user experience for Galaxy users,” highlights a Samsung statement.
Sample Project
Explore a straightforward sample app crafted for Android 14’s foreground service potential, showcasing:
- Proper service initiation with location service type
- Location permission requests
- Activity-bound service updates
- Service cessation from Activity
- Notification permission requests
Final Thoughts
Android 14 demands meticulous attention to foreground service norms, pushing developers towards API 34 compliance. The mandate for service types ensures a regulated, manufacturer-consistent approach.
I hope this guide illuminates your developmental journey. Visit the sample app and further resources to deepen understanding:
- Official Foreground Services Documentation
- Android 14 Service Type Requirements
- Droidcon Presentation on Foreground Services
References: