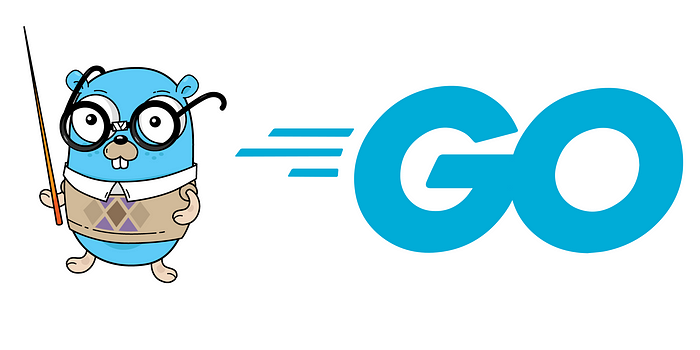
Unlock the Power of Go: Eight Techniques to Transform Your Coding Experience
Imagine limitless potential packed into your Go applications. Yet, the journey to seamless performance is riddled with obstacles most programmers are all too familiar with. The following techniques have guided me through these challenges. It’s time you harnessed them too. Bookmark this article and revisit whenever you need a refresher.
1) Goroutines: A Strategic Deployment
Goroutines open the door to concurrent execution, yet the allure of infinite threading can lead to inefficiency. Every goroutine consumes memory, and excess accumulation can create a bottleneck rather than a boost.
Inefficiency Alert:
for _, item := range bigList {
go process(item)
}
Strategic Limitation:
sem := make(chan struct{}, 100) // Limit to 100 concurrent goroutines
for _, item := range bigList {
sem <- struct{}{}
go func(i Item) {
defer func() { <-sem }()
process(i)
}(item)
}
During a pivotal project aimed at optimizing flight searches across millions of entries, I learned the hard way that goroutines, while efficient, can overwhelm your system if unchecked. Introducing a system to monitor and adjust their number to align with performance needs is crucial.
2) Channels: Handle with Care
Channels enable powerful communication between goroutines but can introduce unexpected waits if unbuffered.
Common Pitfall:
ch := make(chan int)
Optimized Approach:
ch := make(chan int, 100) // Buffer size of 100
Buffered channels accommodate sender operations when receivers lag, mitigating delays and enhancing smooth data flow, especially under heavy workloads.
3) The Perils of Global Variables
Global variables might seem like a shortcut, but they often spawn complex debugging nightmares and obscure code behavior.
Rethink This Habit:
var counter int
func increment() {
counter++
}
Adopt This Practice:
func increment(counter int) int {
return counter + 1
}
counter := 0
counter = increment(counter)
Transitioning my practices away from globals enhanced both clarity and efficiency, minimizing hidden state changes.
4) Slice Mastery: Efficiency in Detail
Slices, ubiquitous in Go, require careful handling to prevent unnecessary data replication.
Optimize Data Management:
// Avoid needless copying:
newSlice := oldSlice[:] // Use slicing
When growing slices, predefine their capacity to eliminate redundant allocations.
Capacity Planning:
s := make([]int, 0, 100) // Capacity of 100
This foresight leads to speedier processing by minimizing runtime memory commits.
5) Profiling for Precision
Insight into code performance can unveil surprising inefficiencies. Leverage Go’s built-in profiling tools to uncover such insights.
Integrate a Profiler:
import (
"runtime/pprof"
"os"
)
func main() {
f, _ := os.Create("cpu.prof")
pprof.StartCPUProfile(f)
defer pprof.StopCPUProfile()
// Execute operations
}
Profiling revealed hidden lags within functions I presumed swift, guiding my optimized recalibrations.
6) Harness the Standard Library
Avoid recreating existing solutions; Go’s standard library is teeming with robust, optimized features.
Rethink Reinventing:
import "sort"
sort.Ints(data)
Embrace existing solutions to supercharge both code succinctness and execution efficiency.
7) Efficient Memory Utilization
Time spent on memory allocation impacts performance—particularly with transient objects.
Object Reuse Strategy:
var buffer bytes.Buffer
for i := 0; i < 1000; i++ {
buffer.Reset()
buffer.WriteString("Some data")
process(buffer.Bytes())
}
Regularly recycling memory buffers reduced unnecessary memory churn, enhancing process flow.
8) Maximize with Latest Go Versions
Stay on the cutting edge. Each new Go iteration offers built-in speed and efficiency.
Initially resistant to upgrades, I found that transitioning to newer versions immediately enhanced performance, requiring no alterations.
Conclusion: Craftsmanship in Code
These insights stem from a journey filled with challenges and breakthroughs. Each project demands a bespoke approach, yet mindfulness of these practices can resolve bottlenecks efficiently. Remember: test and profile promptly whenever you implement changes to ensure the optimization hits its mark. Got any remarkable Go tricks? Share them in the comments!
Keep pushing the boundaries of what you can achieve with Go! Happy coding!