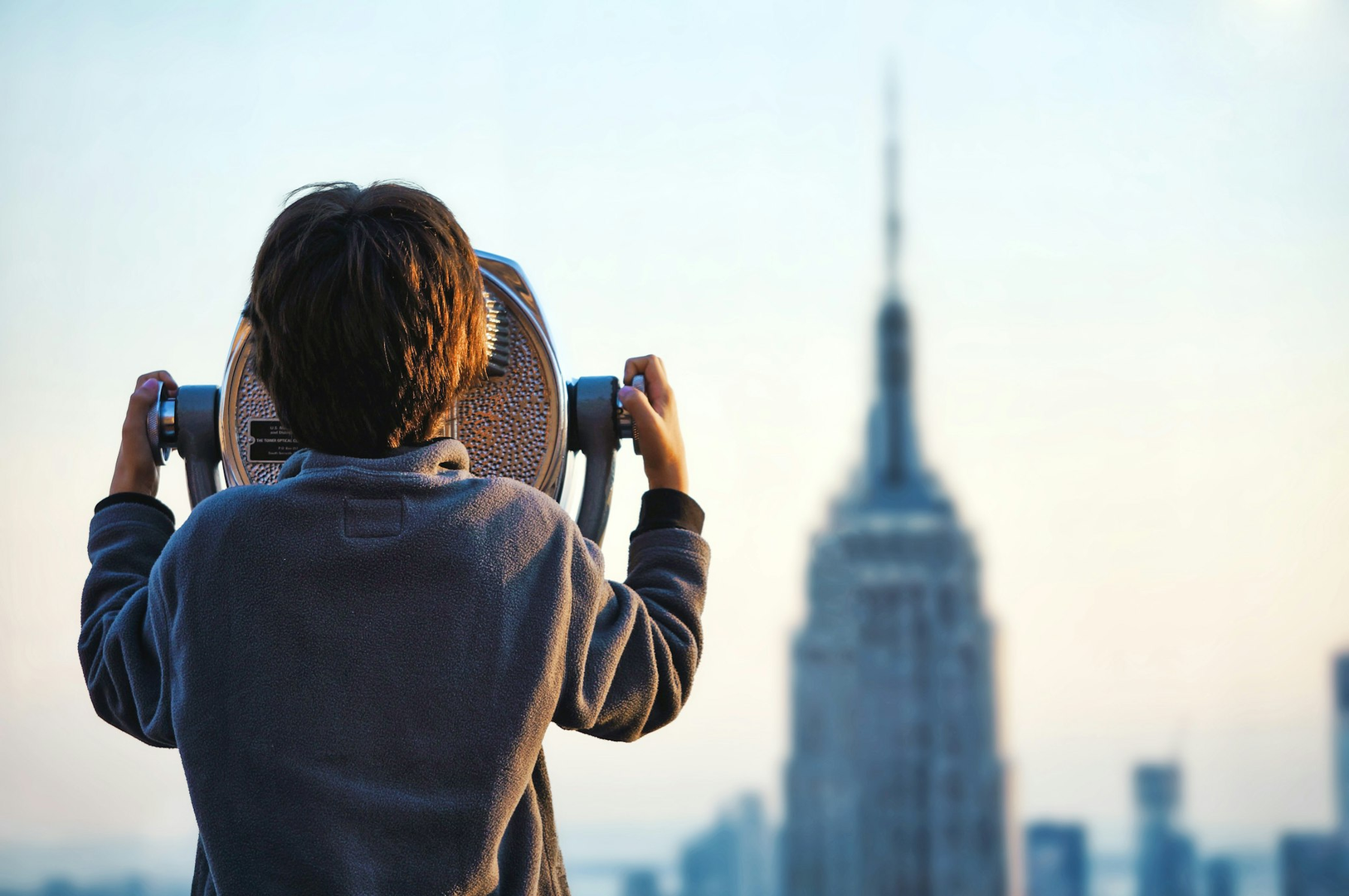
In the evolving realm of React development, the craft of managing state and ensuring seamless communication between components can grow intricate as applications expand in scope. Although Redux and Context API have carved their niche in this space, they often accompany a suite of boilerplate and complexity. But what if we could embrace a more graceful method for handling component interaction, eschewing reliance on these conventional tools? Welcome to the world of event-driven React, powered by the Observer Pattern.
Navigating the Maze: Prop Drilling and State Management Challenges
Within React lies the complexity of prop drilling, a situation where data or callbacks must traverse numerous component layers to reach a distant child. This not only makes your scripts cumbersome but also increases the potential for errors. Although tools like Redux and Context API are designed to centralize state management, they bring their own set of drawbacks:
- Redux frequently demands substantial boilerplate code, which can seem like a burden for smaller applications.
- Context API offers simplicity but requires cautious handling to avoid unnecessary re-renders.
While alternatives such as Zustand exist to counter Redux, these are external libraries, and the quest here is to resolve prop drilling with nothing but native solutions. Imagine achieving component interaction more independently without leaning on these tools.
Embracing the Observer Pattern: A Streamlined Alternative
The Observer Pattern emerges as a design philosophy where an entity (the subject) keeps track of its dependents (observers) and informs them when state changes. This model is ideal for crafting an event bus—a centralized communication hub where components can effortlessly publish and subscribe to events.
Utilizing an event bus, components communicate directly, bypassing the tedious prop-passing or the need for a centralized state management library. This strategy fosters loose coupling and renders your code base more modular and maintainable.
Imagine the architecture:
Subject (Event Bus): Features methods like on()
, off()
, and emit()
.
Observers (Components): Represented as boxes for React components (Button
, MessageDisplay
). The Button initiates an event.
Event Flow (Arrows): Connecting Button
with the Event Bus (via emit('buttonClicked')
) and from the Event Bus to MessageDisplay
(via on('buttonClicked')
).
Crafting an Event Bus in React
Let’s delve into the simplicity of creating an event bus utilizing the Observer Pattern. Here’s your guide:
1. Construct the Event Bus
Begin by defining a straightforward event bus class:
class EventBus {
constructor() {
this.listeners = {};
}
on(event, callback) {
if (!this.listeners[event]) {
this.listeners[event] = [];
}
this.listeners[event].push(callback);
}
off(event, callback) {
if (this.listeners[event]) {
this.listeners[event] = this.listeners[event].filter(
(listener) => listener !== callback
);
}
}
emit(event, data) {
if (this.listeners[event]) {
this.listeners[event].forEach((listener) => listener(data));
}
}
}
const eventBus = new EventBus();
export default eventBus;
The EventBus
class bestows upon components the power to subscribe to events (on
), withdraw subscriptions (off
), and project events (emit
).
2. Integrating the Event Bus into Components
With our event bus standing ready, let’s witness its application within React components.
Publishing Events
Components can herald an event using the emit
method:
import React from 'react';
import eventBus from './eventBus';
const Button = () => {
const handleClick = () => {
eventBus.emit('buttonClicked', { message: 'Button was clicked!' });
};
return <button onClick={handleClick}>Click Me</button>;
};
export default Button;
Subscribing to Events
Another component might enroll in the event by using the on
method:
import React, { useEffect, useState } from 'react';
import eventBus from './eventBus';
const MessageDisplay = () => {
const [message, setMessage] = useState('');
useEffect(() => {
const handleButtonClick = (data) => {
setMessage(data.message);
};
eventBus.on('buttonClicked', handleButtonClick);
return () => {
eventBus.off('buttonClicked', handleButtonClick);
};
}, []);
return <div>{message}</div>;
};
export default MessageDisplay;
Here, the MessageDisplay
component updates its state when the button is clicked, displaying the message transmitted from the Button
component.
The Importance of Testing the Event Bus
The event bus forms the backbone of our architecture. It is vital to ensure its functionality through robust testing. We will employ unit tests to verify the EventBus
class’s ability to manage subscriptions, unsubscriptions, and event projections accurately.
Example Test Suite for EventBus
:
import EventBus from './eventBus';
describe('EventBus', () => {
let eventBus;
beforeEach(() => {
eventBus = new EventBus();
});
it('should subscribe to an event and call the listener when the event is emitted', () => {
const listener = jest.fn();
eventBus.on('testEvent', listener);
eventBus.emit('testEvent', { message: 'Hello, world!' });
expect(listener).toHaveBeenCalledWith({ message: 'Hello, world!' });
});
it('should unsubscribe from an event and not call the listener', () => {
const listener = jest.fn();
eventBus.on('testEvent', listener);
eventBus.off('testEvent', listener);
eventBus.emit('testEvent', { message: 'Hello, world!' });
expect(listener).not.toHaveBeenCalled();
});
it('should handle multiple listeners for the same event', () => {
const listener1 = jest.fn();
const listener2 = jest.fn();
eventBus.on('testEvent', listener1);
eventBus.on('testEvent', listener2);
eventBus.emit('testEvent', { message: 'Hello, world!' });
expect(listener1).toHaveBeenCalledWith({ message: 'Hello, world!' });
expect(listener2).toHaveBeenCalledWith({ message: 'Hello, world!' });
});
it('should not throw an error when emitting an event with no listeners', () => {
expect(() => {
eventBus.emit('testEvent', { message: 'Hello, world!' });
}).not.toThrow();
});
});
Component Testing for Event Bus Utilization
Testing components that engage with the event bus is equally crucial. Employ React Testing Library along with Jest to certify that components seamlessly publish and subscribe to events.
Example: Testing the Button
Component
The Button
component’s task is to dispatch an event on click. Let’s validate that this operation occurs correctly:
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
import eventBus from './eventBus';
jest.mock('./eventBus'); // Mock the event bus
describe('Button', () => {
it('should emit an event when clicked', () => {
const { getByText } = render(<Button />);
const button = getByText('Click Me');
fireEvent.click(button);
expect(eventBus.emit).toHaveBeenCalledWith('buttonClicked', {
message: 'Button was clicked!',
});
});
});
Example: Testing the MessageDisplay
Component
The MessageDisplay
component listens for events and updates its state accordingly. Our goal here is to confirm that it reacts to event emissions as anticipated:
import React from 'react';
import { render, act } from '@testing-library/react';
import MessageDisplay from './MessageDisplay';
import eventBus from './eventBus';
jest.mock('./eventBus'); // Mock the event bus
describe('MessageDisplay', () => {
it('should update the message when an event is received', () => {
const { getByText } = render(<MessageDisplay />);
// Simulate the event being emitted
act(() => {
eventBus.listeners['buttonClicked'][0]({ message: 'Button was clicked!' });
});
expect(getByText('Button was clicked!')).toBeInTheDocument();
});
it('should clean up the event listener on unmount', () => {
const { unmount } = render(<MessageDisplay />);
unmount();
expect(eventBus.off).toHaveBeenCalledWith(
'buttonClicked',
expect.any(Function)
);
});
});
Key Testing Strategies
- Mock the Event Bus: Use
jest.mock
to simulate the event bus and manage its actions in your tests. - Inspect Event Emission: Confirm that components broadcast the correct events with precise data.
- Verify Event Subscription: Validate that components respond appropriately to events.
- Ensure Cleanup: Make sure event listeners are deactivated upon component unmounting to avert memory leaks.
Tools for Robust Testing
- Jest: Utilized for unit testing and mocking.
- React Testing Library: Facilitates testing of React components, mirroring user interactions closely.
- Mock Functions: Employ
jest.fn()
to simulate event listeners and assess their actions.
Choosing an Event Bus: The Multifaceted Advantages
Embracing an event bus yields several benefits:
- Decoupled Components: Entities no longer need awareness of each other, fostering separation of concerns through the event bus.
- Elimination of Prop Drilling: Free yourself from passing props across multiple component layers.
- Lightweight Structure: Compared to Redux or Context, an event bus is easy to implement and free from additional dependencies.
- Scalable Design: Gracefully accommodate app growth by adjusting events and listeners without escalating complexity.
Ideal Scenarios for Event Bus Application
While powerful, the event bus serves specific contexts best. Here’s where it excels:
- Small to Medium Applications: In smaller projects, it can serve as a versatile alternative to Redux or Context.
- Cross-Component Communication: Perfect for facilitating interaction across diverse component hierarchies.
- Decoupled Logic: Facilitates maintainable, reusable components by supporting autonomy.
Yet, in expansive applications requiring sophisticated state management, Redux or Context might still reign supreme.
Concluding Thoughts
The Observer Pattern, alongside an event-driven approach, offers a refined and sophisticated path for handling component communication within React. By deploying an event bus, you negate the burdens of prop drilling, slash boilerplate, and champion a more decoupled, maintainable component ecosystem.
Not a universal substitute for every state-management situation, the event bus is nonetheless an indispensable asset in any React developer’s toolkit. Explore it in your forthcoming project—a tool primed to simplify and elevate your code!