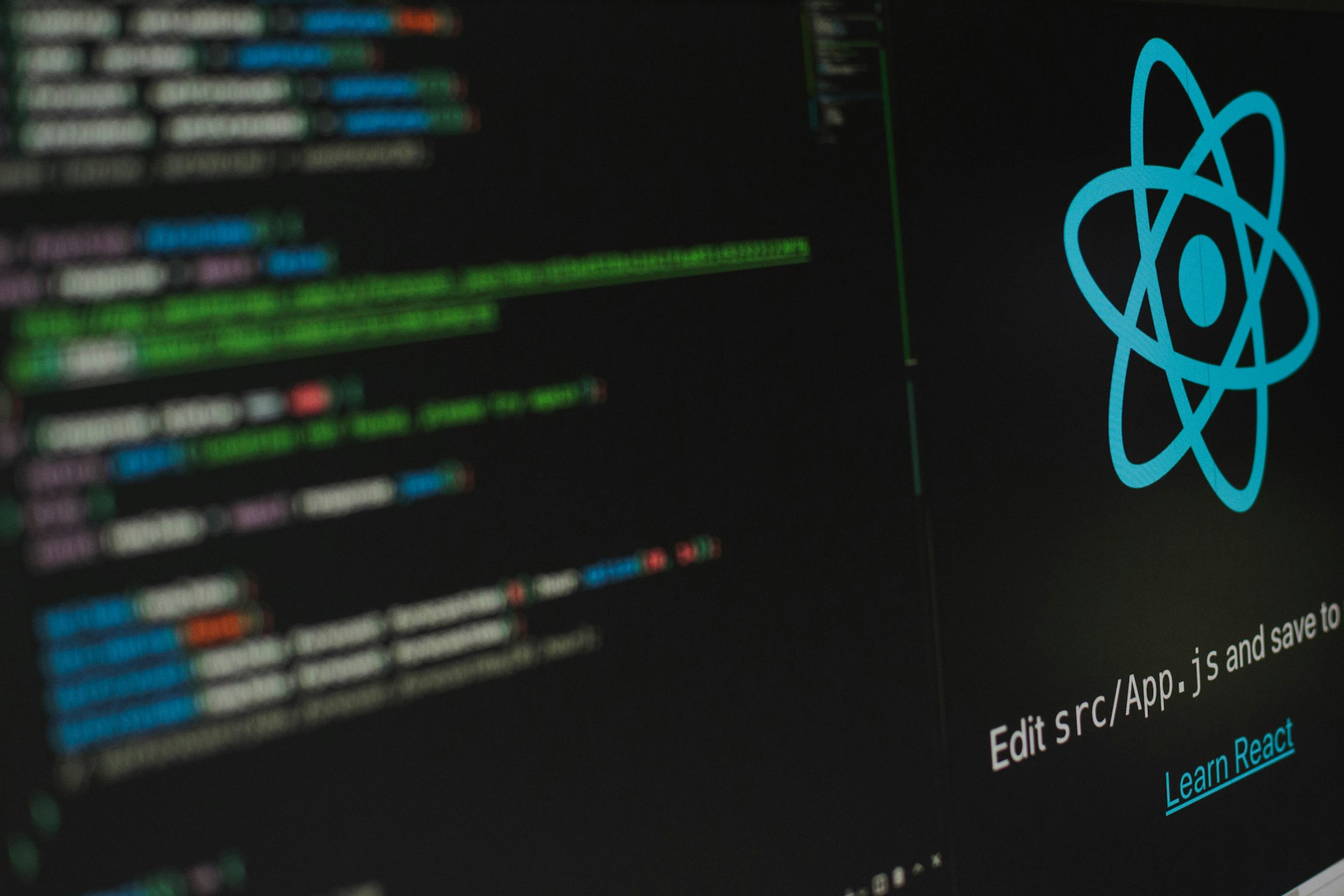
Introduction
In the dynamic landscape of software development, efficiency isn’t just a luxuryâit’s a necessity. During a recent project, I encountered a challenge with an outdated React.js import strategy. Join me on this enlightening journey where we transform the mundane into efficient with a forward-thinking twist: the Wrapper Pattern.
The Challenge in Focus
In the throes of this project, I noticed a common yet inefficient practice: importing Framer Motion like this:
import { motion } from 'framer-motion' // leads to a hefty import size of 110.63KB
Unveiling the Problem
While 110.63KB seems trivial at first glance, it poses a significant heft when widespread. Consider thisâimporting it across multiple components will expand your bundle size exponentially. In a scenario where it appears in 7 components, you’re looking at:
7 x 110.63KB = 774.41KB
Imagine that your entire application stacks up to a mere 1.5MBâFramer Motion alone might gorgeously consume 51.3% of it. That’s a startlingly disproportionate share for animations!
Understanding the Core of the Issue
- Tree Shaking Limitations â Framer Motion exports an expansive smorgasbord of utilities, which means importing it in bulk inadvertently tugs along unwanted code.
- Redundant Imports Across Files â Although only a single instance features in the final bundle, unwieldy imports bloat your application.
The Solution Unveiled
Enter our hero: the MotionWrapper.tsx
file.
// A more concise solution to circumvent large imports
import { m } from 'framer-motion'
const MotionWrapper = m;
export default MotionWrapper;
Notably, Framer Motion offers {m}
, a lightweight version incorporating essentials. Let’s now see how this reimagined approach impacts your project.
Visualize this simpler transformation:
// App.tsx
import { motion } from 'framer-motion';
const App = () => {
return (
<motion.div {props} />
);
}
Refactor seamlessly to:
// App.tsx
import { MotionWrapper } from '@/components/MotionWrapper';
const App = () => {
return (
<MotionWrapper.div {props} />
);
}
Visual Finesse
Your streamlined build now manifests as:
This shift visualized with vite-bundle-visualizer showcases your path from chaos to clarity through optimization.
Extending the Approach: The Lodash Perspective
A similar tale unfolds with Lodash when you go from old-school:
import _ from 'lodash'; // imports entire library bloating bundle size
To a tailored, efficient model with LodashWrapper:
// LodashWrapper.tsx
import debounce from 'lodash/debounce';
import throttle from 'lodash/throttle';
export const LodashWrapper = { debounce, throttle };
Rather than dragging the entire library, import specifics:
import { LodashWrapper } from '@/utils/LodashWrapper';
const handleInput = LodashWrapper.debounce((value) => {
console.log('Debounced Input:', value);
}, 300);
Enlightened Conclusion
We’ve achieved a reduced bundle size around 50KB
, a paltry 3%
of our total package. This strategy not only streamlines but future-proofs your code landscape.
Navigating Library Selection with Insight
Selecting libraries demands a discerning eye. Contemplate their import strategiesâcritical when choosing between offerings like Recharts
and Chart.js
.
Recharts requires a holistic library import, consequently bloating bundle size:
import { LineChart } from 'recharts'; // pulls in unnecessary code
In contrast, Chart.js provides nimble, treeshakable imports, allowing you to cherry-pick required elements:
import { Chart, LineElement, CategoryScale } from 'chart.js';
Chart.register(LineElement, CategoryScale);
Such flexibility makes Chart.js a prime choice for those keen on performance.
Footnotes:
- Highlighting Recharts isn’t an indictment but a pragmatic illustration.
- Anticipate Recharts addressing these concerns in its forthcoming
v3
iteration